Sending activities
On this page you will learn how to send activities using your bot framework.
This page also describes how activityParams
and sessionParams
properties are sent.
Refer to Changing call settings for more information.
AudioCodes Bot API
See Introduction for full description of the syntax of activities.
To summarize, activities should be sent as displayed throughout this guide, with the
addition of the attributes
id
and timestamp
.
For example:
{
"id": "59f09f09-feb6-40d4-8852-b960502d9841",
"timestamp": "2020-10-18T15:22:35.211Z",
"type": "event",
"name": "hangup"
}
If you want to include activityParams
or sessionParams
properties, you
should
just add
them to the activity. For example:
{ "id": "4ddc321e-6888-451f-9014-9446ec22d64d", "timestamp": "2020-10-18T15:27:12.837Z", "type": "event", "name": "hangup", "activityParams": { "hangupReason": "completed successfully" } }
Microsoft Bot Framework
Activities are natively supported on Microsoft Bot Framework, so you can send them as described in the Bot Framework documentation.
If you want to include activityParams
or sessionParams
properties, you
should place them inside a channelData
property. For example:
{ "type": "event", "name": "hangup", "channelData": { "activityParams": { "hangupReason": "completed successfully" } } }
Microsoft Copilot Studio
Activity and session params can be sent with message to the user (Sending messages).
The following sample shows a custom payload sent from composer to VoiceAI Connect:
{ "activityParams": { "<parameter name 1>": "<parameter value>", "<parameter name 2>": "<parameter value>" } }
Microsoft Copilot Studio legacy
Activities are sent via bot Composer Framework see Sending actions to VoiceAI Connect on how to send activities.
The following sample shows a custom payload sent from composer to VoiceAI Connect:
[Activity type = event name = <event name> channelData = ${json(' { "activityParams": { "<parameter name 1>": "<parameter value>", "<parameter name 2>": "<parameter value>" } } ')} ]
Google Dialogflow
The messages sent by a Dialogflow agent are based on intent’s responses (on Dialogflow CX, responses are called fulfillment), which have a different syntax than that of the activities described throughout this guide. To overcome this, VoiceAI Connect uses two approaches, both of which can be used by the bot.
The first approach is using native Dialogflow responses. VoiceAI Connect automatically
translates supported responses into activities. For example, a Text Response is translated into a message
activity (for playing the text to the user). As another example, an intent
that
is marked to end the conversation is translated into a hangup
activity.
The second approach is for the bot to send activities according to VoiceAI Connect syntax. For
doing this, a Custom Payload response with an activities
property should be added
(see
below). The value of the activities
property should contain an array of activities
to
be executed by VoiceAI Connect.
activities
property will be discarded.
The following example shows a Custom Payload response for performing the hangup
activity:
{ "activities": [ { "type": "event", "name": "hangup" } ] }
If you want to include activityParams
or sessionParams
properties, you
should
add
them to the activity. For example:
{ "activities": [ { "type": "event", "name": "hangup", "activityParams": { "hangupReason": "completed successfully" } } ] }
How to add Custom Payload responses?
As described above, Custom Payload responses are used for sending activities by the bot.
For advanced usages, Custom Payload responses could also be used for setting
activityParams
for the native Dialogflow response and sessionParams
which would be applied to the session
outside the scope of an activity.
Documentation regarding Custom Payload responses can be found in the following links:
The following table lists the properties that are supported on Custom Payload responses:
Property | Description |
---|---|
activities |
Array of activities to be executed by VoiceAI Connect. The activities will be executed after any native Dialogflow responses. |
activityParams | Parameters applied to the native Dialogflow response. |
sessionParams | Parameters applied to the whole session. |
The following example shows how Custom Payload can be configured through the Dialogflow user interface:
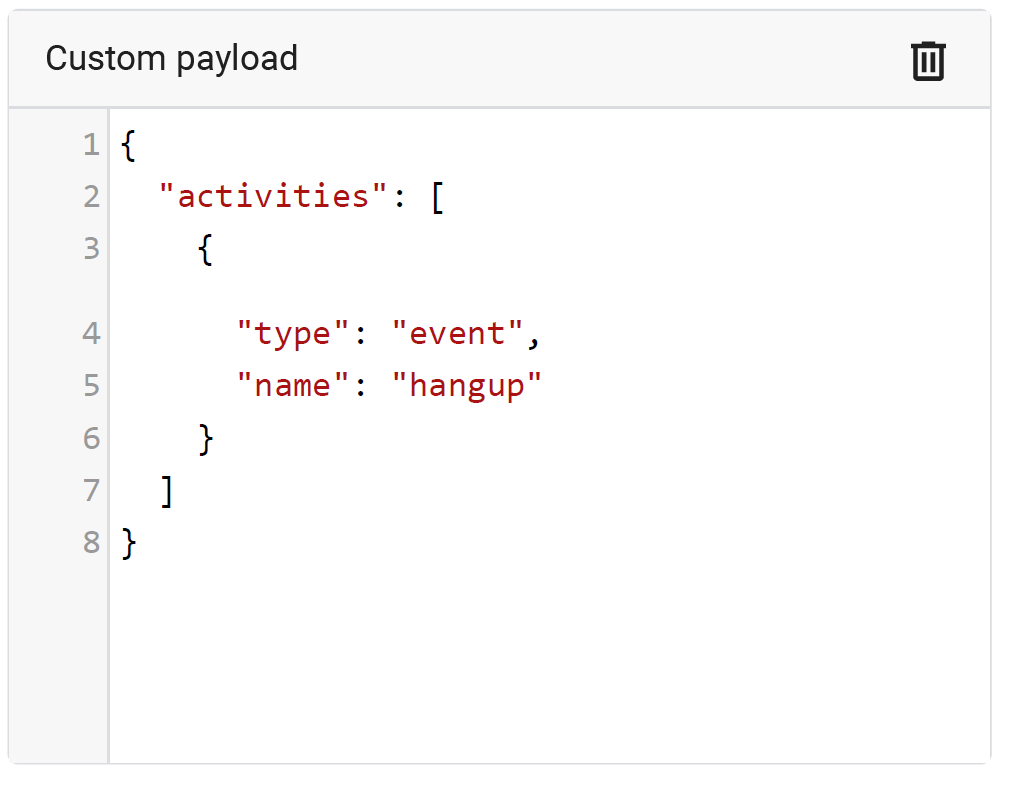
Dialogflow Webhook examples
This section provides advanced examples of using Dialogflow ES webhooks for sending activities.
This is an example of handling text messages and performing sendMetadata
:
function fallback(agent) { const participant = request.body.originalDetectIntentRequest.payload.parameters.participant; agent.add(new Payload('PLATFORM_UNSPECIFIED', { activities: [ { name: 'sendMetaData', type: 'event', value: { participant: participant, text: request.body.queryResult.queryText } } ] }, { rawPayload: true, sendAsMessage: true })); }
This is an example of a webhook that handles the WELCOME
event and
performs startRecognition
to all participants:
function welcome(agent) { const activities = request.body.queryResult.outputContexts.find( (c) => c.name.endsWith('welcome')).parameters.participants.map( (p) => ({ activityParams: { targetParticipant: p.participant }, name: 'startRecognition', type: 'event' })); const payload = new Payload( 'PLATFORM_UNSPECIFIED', { activities }, { rawPayload: true, sendAsMessage: true } ); agent.add(payload); }